Create Rebar Data#
- class opstool.preprocessing.Rebars[source]#
A class to create the rebar point.
- add_rebar_circle(xo, radius, dia, gap=0.1, n=None, angle1=0.0, angle2=360, matTag=None, color='black', group_name=None)[source]#
Add the rebars along a circle.
Parameters#
- xo: list[float, float]
Center coords of circle, [(xc, yc)].
- radius: float
Radius of circle.
- dia: float
rebar dia.
- gap: float, default=0.1
Rebar space.
- n: int, default=None
The number of rebars, if not None, update the Arg gap according to n. This means that if you know the number of rebars, you don’t need to input gap or set gap to any number.
- angle1: float
The start angle, degree.
- angle2: float
The end angle, deree.
- matTag: int
OpenSees material matTag for rebar previously defined.
- color: str or rgb tuple.
Color to plot rebar.
- group_name: str
Assign rebar group name.
Returns#
None
- add_rebar_line(points, dia, gap=0.1, n=None, closure=False, matTag=None, color='black', group_name=None)[source]#
Add rebar along a line, can be a line or polygon.
Parameters#
- points: list[list[float, float]]
A list of rebar coords, [(x1, y1), (x2, y2),…,(xn, yn)], in which each element represents a corner point, and every two corner points are divided by the arg gap.
- dia: float
Rebar dia.
- gap: float, default=0.1
Rebar space.
- n: int, default=None
The number of rebars, if not None, update the Arg gap according to n. This means that if you know the number of rebars, you don’t need to input gap or set gap to any number.
- closure: bool, default=False
If True, the rebar line is a closed loop.
- matTag: int
OpenSees mat Tag for rebar previously defined.
- color: str or rgb tuple.
Color to plot rebar.
- group_name: str
Assign rebar group name
Returns#
None
Example#
import opstool as opst
outlines = [[0, 0], [2, 0], [2, 2], [0, 2]]
coverlines = opst.offset(outlines, d=0.05)
cover = opst.add_polygon(outlines, holes=[coverlines])
holelines = [[0.5, 0.5], [1.5, 0.5], [1.5, 1.5], [0.5, 1.5]]
core = opst.add_polygon(coverlines, holes=[holelines])
sec = opst.SecMesh()
sec.assign_group(dict(cover=cover, core=core))
sec.assign_mesh_size(dict(cover=0.02, core=0.05))
sec.assign_group_color(dict(cover="gray", core="green"))
sec.mesh()
Add Rebars:
# add rebars
rebars = opst.Rebars()
rebar_lines1 = opst.offset(outlines, d=0.05 + 0.032 / 2)
rebars.add_rebar_line(
points=rebar_lines1, dia=0.032, gap=0.1, color="red",
)
rebar_lines2 = opst.offset(holelines, d=-(0.05 + 0.02 / 2))
rebars.add_rebar_line(
points=rebar_lines2, dia=0.020, gap=0.1, color="black",
)
rebar_lines3 = [[0.3, 0.3], [1.7, 0.3], [1.7, 1.7], [0.3, 1.7]]
rebars.add_rebar_line(
points=rebar_lines3, dia=0.026, gap=0.15, closure=True,
color="blue",
)
# add to the sec
sec.add_rebars(rebars)
Visualization:
sec.get_sec_props(display_results=False, plot_centroids=False)
sec.centring()
sec.view(fill=True, engine='mpl')
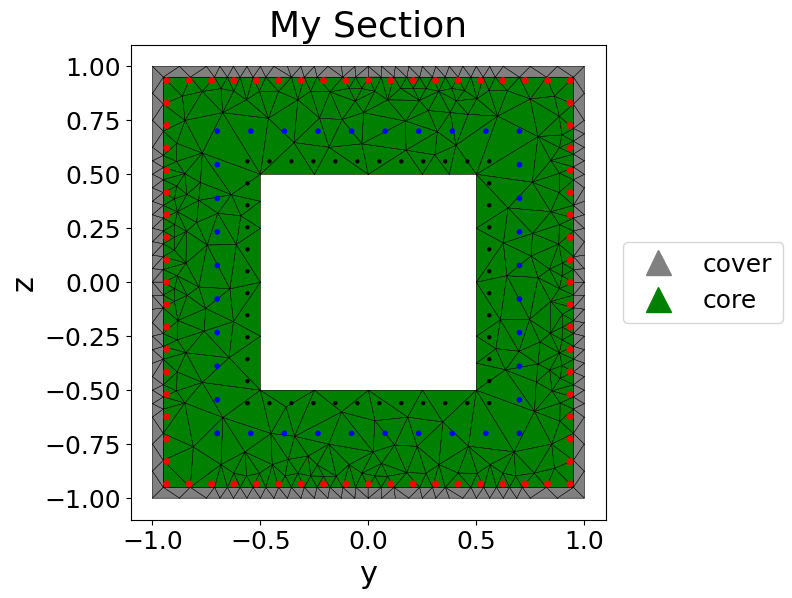